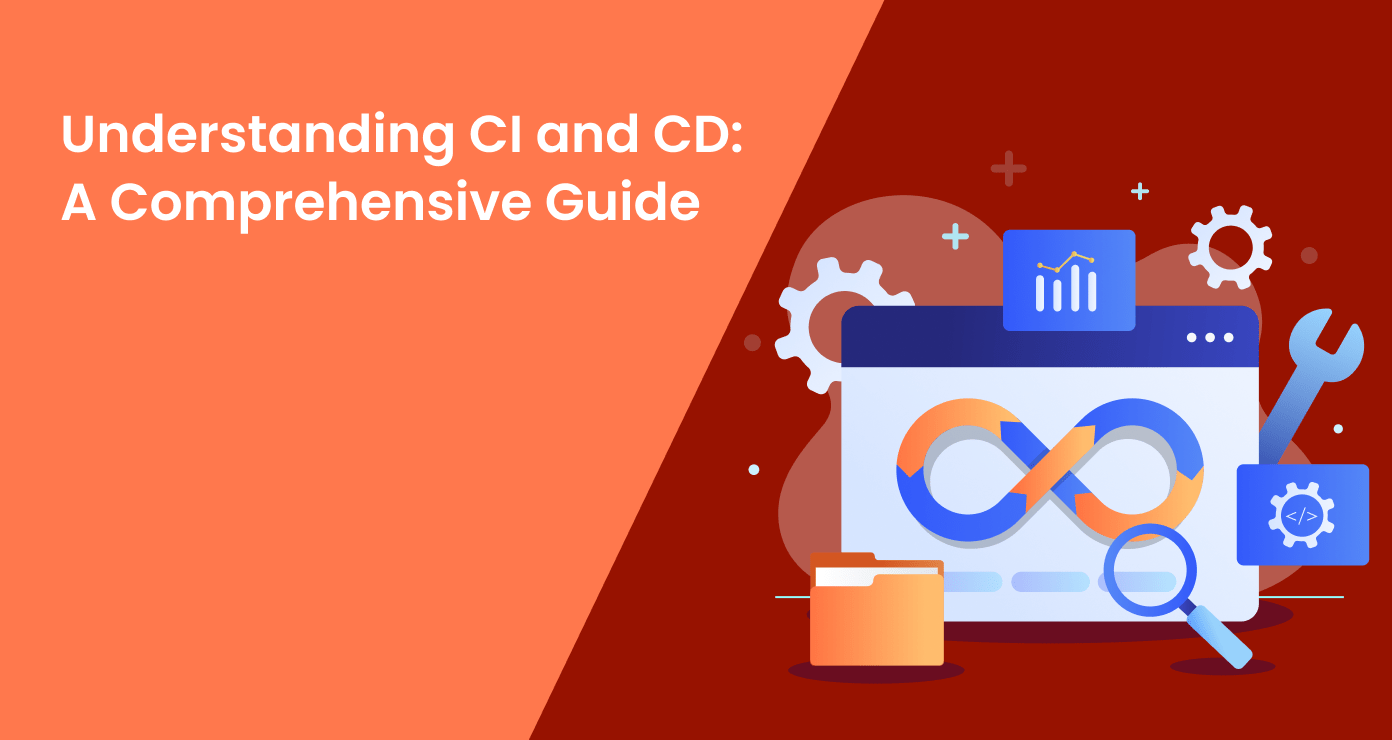
In the rapidly evolving world of software development, Continuous Integration (CI) and Continuous Delivery (CD) have emerged as essential practices for teams striving to deliver high-quality software efficiently.
This comprehensive guide delves into the intricacies of CI and CD, exploring their definitions, benefits, and implementation strategies. We’ll examine popular CI server tools, with a special focus on CI and CD with Jenkins, and discuss best practices that can help development teams streamline their workflows. From understanding the basics to exploring future trends, this article aims to equip you with the knowledge needed to leverage CI and CD effectively in your software development projects.
What are CI and CD?
Continuous Integration (CI)
CI is a development practice where developers integrate code into a shared repository frequently, usually several times a day. Each integration is verified by an automated build and automated tests. This approach helps detect errors quickly and locate them more easily.
The main goals of CI are to:
- Improve code quality by catching integration issues early
- Reduce the time between writing code and getting feedback
- Ensure that the software is always in a releasable state
CI typically involves the following steps:
- Developers commit code changes to a version control system
- The CI server tools detect the change and trigger a build
- The code is compiled and unit tests are run
- If the build or tests fail, the team is notified immediately
- Developers fix any issues promptly to keep the main branch stable
Continuous Delivery (CD)
CD extends CI by automatically deploying all code changes to a testing or production environment after the build stage. This means that on top of automated testing, you have an automated release process and can deploy your application at any point in time by clicking a button.
CD aims to:
- Reduce the time between writing code and getting it to users
- Ensure that deploying software is a reliable, low-risk operation
- Provide faster feedback from users
The CD pipeline typically includes:
- Automated deployment to staging environments
- Automated acceptance and performance tests
- Manual approval for production deployment
- Automated deployment to production
(Source: Atlassian, atlassian.com)
Continuous Deployment
Sometimes, CD is taken a step further into Continuous Deployment. In this practice, every change that passes all stages of your production pipeline is released to your customers. There’s no human intervention, and only a failed test will prevent a new change from being deployed to production.
The Relationship Between CI and CD
CI and CD are complementary practices that work together to improve the software delivery process:
- CI ensures that code changes are regularly built, tested, and merged to a shared repository
- CD takes the validated code from CI and automates the delivery to staging and production environments
Together, they form a CI/CD pipeline that streamlines the entire software development lifecycle, from code changes to production deployment.
By implementing CI and CD, teams can:
- Deliver updates to users more frequently and reliably
- Reduce manual processes and human error
- Improve collaboration between development and operations teams
- Increase confidence in the codebase and deployment process
In the next sections, we’ll explore how these practices are implemented using popular CI server tools, with a focus on CI and CD with Jenkins.
CI Server Tools: An Overview
CI server tools are essential for implementing CI/CD pipelines. They automate the integration and testing processes. Let’s explore some popular CI tools in more detail:
Jenkins
Jenkins is one of the most widely used open-source CI tools. It offers:
- Extensive plugin ecosystem with over 1,800 plugins
- Support for distributed builds across multiple machines
- Easy configuration through web interface
- Pipelines as code using Jenkinsfile
- Integration with various version control systems and build tools
Jenkins is highly customizable and can be used for projects of any size. Its flexibility makes it a popular choice for many organizations.
GitLab CI
GitLab CI is part of the GitLab platform, offering:
- Tight integration with GitLab’s version control system
- Built-in Docker support for consistent build environments
- Auto DevOps for automatic CI/CD configuration
- Kubernetes integration for easy deployment
- Parallel execution of jobs for faster builds
GitLab CI’s main advantage is its seamless integration with GitLab, providing a single platform for the entire DevOps lifecycle.
Travis CI
Travis CI is known for its simplicity and is popular among open-source projects. It features:
- Easy setup with .travis.yml configuration file
- Built-in support for many programming languages
- Free for open-source projects
- Automatic testing on multiple platforms (Linux, macOS, Windows)
- Integration with GitHub and Bitbucket
Travis CI’s simplicity and cloud-based nature make it an excellent choice for projects that don’t require complex build environments.
CircleCI
CircleCI offers fast builds and easy parallelization of tests. Key features include:
- First-class Docker support
- Workflows for complex job orchestration
- SSH access to build containers for debugging
- Caching mechanisms to speed up builds
- Insights and analytics on build performance
CircleCI’s strength lies in its speed and ability to handle complex pipelines efficiently.
TeamCity
TeamCity is a CI server by JetBrains, offering:
- Intuitive user interface
- Powerful build chain feature for complex dependencies
- Built-in test reporting and code coverage
- Integration with JetBrains IDEs
- Customizable with plugins and REST API
TeamCity provides a user-friendly experience while offering advanced features for larger enterprises.
Comparing CI Server Tools
When choosing a CI tool, consider:
- Integration with existing tools and workflows
- Scalability for your project size
- Ease of use and learning curve
- Support for your technology stack
- Cost (open-source vs. commercial)
Each tool has its strengths:
- Jenkins excels in flexibility and customization
- GitLab CI offers an all-in-one DevOps platform
- Travis CI is great for open-source projects
- CircleCI shines in speed and parallelization
- TeamCity provides a polished, enterprise-ready solution
The choice of CI tool often depends on specific project requirements and team preferences. Many organizations use multiple tools across different projects to leverage the strengths of each.
In the following sections, we’ll dive deeper into CI and CD with Jenkins, as it remains one of the most versatile and widely adopted tools in the industry.
CI and CD with Jenkins
What is Jenkins?
Jenkins is an open-source automation server that helps automate parts of software development related to building, testing, and deploying, facilitating CI and CD. CI and CD with Jenkins has become increasingly popular due to its flexibility and extensive plugin ecosystem.
(Source: Jenkins Developer Documentation, jenkins.io/doc/developer/)
Setting Up Jenkins
To set up Jenkins:
- Download and install Jenkins on your server.
- Configure Jenkins through its web interface.
- Install necessary plugins for your project needs.
Creating a CI Pipeline with Jenkins
A basic CI pipeline in Jenkins might include:
- Source Control Management (SCM) checkout
- Compile the code
- Run unit tests
- Run integration tests
- Generate reports
Here’s a simple Jenkinsfile example for CI and CD with Jenkins:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'mvn deploy'
}
}
}
}
This pipeline builds, tests, and deploys a Maven project, demonstrating a basic CI and CD with Jenkins setup.
Benefits of CI and CD
Implementing CI and CD offers numerous advantages:
- Faster time to market
- Improved code quality
- Reduced deployment risks
- Increased developer productivity
- Better collaboration among team members
Best Practices for CI and CD
To get the most out of CI and CD, consider implementing these best practices:
1. Commit code frequently
Encourage developers to commit small changes often, ideally several times a day. This practice:
- Reduces the risk of merge conflicts
- Makes it easier to identify and fix issues
- Promotes a steady flow of updates
2. Write automated tests
Develop a comprehensive suite of automated tests, including:
- Unit tests for individual components
- Integration tests for interactions between components
- End-to-end tests for full system functionality
Aim for high test coverage to catch bugs early and ensure code quality.
3. Fix broken builds immediately
When a build fails:
- Make fixing it the top priority
- Avoid adding new features until the build is fixed
- Use build status indicators to keep the team informed
This practice maintains a stable main branch and prevents compounding issues.
4. Keep the build fast
Optimize your build process to run quickly:
- Parallelize tests where possible
- Use incremental builds
- Implement efficient caching strategies
Aim for builds that complete in less than 10 minutes to maintain developer productivity.
5. Test in a clone of the production environment
Ensure your staging environment closely mirrors production:
- Use the same operating systems, libraries, and configurations
- Implement data anonymization for realistic testing
- Regularly sync staging with production configurations
This practice reduces “works on my machine” issues and improves deployment reliability.
6. Make deployments easy
Automate the deployment process:
- Use infrastructure as code for consistent environments
- Implement feature flags for controlled rollouts
- Develop rollback procedures for quick recovery
Easy deployments reduce stress and encourage more frequent releases.
7. Version control everything
Keep all project assets in version control:
- Source code
- Configuration files
- Build scripts
- Database schemas
- Documentation
This ensures traceability and makes it easier to reproduce any version of your application.
8. Implement trunk-based development
Encourage working on the main branch or short-lived feature branches:
- Merge feature branches quickly (within a day or two)
- Use feature flags for longer-term work
- Practice continuous code review
This approach reduces merge conflicts and keeps the codebase integrated.
9. Monitor and optimize the pipeline
Regularly review your CI/CD pipeline:
- Track key metrics like build time, success rate, and deployment frequency
- Identify and address bottlenecks
- Continuously refine and improve the process
An optimized pipeline leads to faster, more reliable deliveries.
(Source: Spiceworks, spiceworks.com/tech/devops/)
10. Secure your CI/CD pipeline
Implement security measures throughout your pipeline:
- Use secrets management for sensitive information
- Implement access controls and audit logging
- Scan for vulnerabilities in code and dependencies
- Validate artifacts before deployment
A secure pipeline protects your code and infrastructure from potential threats.
This cultural shift ensures everyone is invested in the success of your CI/CD practices.
By following these best practices, teams can maximize the benefits of CI and CD, leading to faster, more reliable software delivery. Remember that implementing these practices is an iterative process. Start with the most critical practices for your team and gradually incorporate others as you mature your CI/CD processes, whether you’re using CI and CD with Jenkins or other CI server tools.
Challenges in Implementing CI and CD
While beneficial, implementing CI and CD can be challenging:
- Cultural resistance to change
- Initial setup time and cost
- Maintaining test suites
- Ensuring security in automated deployments
These challenges can be particularly pronounced when setting up CI and CD with Jenkins or other CI server tools for the first time. However, with proper planning and commitment, these hurdles can be overcome.
CI and CD in Different Development Methodologies
CI and CD align well with various development methodologies:
Agile Development
CI and CD support Agile’s focus on iterative development and frequent releases. CI tools like Jenkins can be integrated into Agile workflows to automate testing and deployment processes.
Note:
Learn more about Agile development in our other article Agile Software Development: Metrics, Programs, Values and Principles.
DevOps
CI and CD are core practices in DevOps, bridging development and operations. CI server tools play a crucial role in implementing DevOps principles by automating the build, test, and deployment pipeline.
Note:
Check why the IT department should take part in the cloud cost optimization process in our other article FinOps vs DevOps Approach to Optimizing Costs.
Future Trends in CI and CD
The landscape of CI and CD is constantly evolving. Here are some key trends shaping the future of these practices:
AI-powered testing and deployment
Artificial Intelligence (AI) and Machine Learning (ML) are revolutionizing CI/CD processes:
- Predictive analytics: AI can analyze historical data to predict potential failures or bottlenecks in the pipeline.
- Automated test generation: ML algorithms can generate test cases based on code changes and past bugs.
- Intelligent monitoring: AI-powered tools can detect anomalies in production and automatically trigger rollbacks or fixes.
- Self-healing systems: ML models can learn from past incidents to automatically resolve common issues without human intervention.
Increased use of containerization
Containerization, especially with Docker and Kubernetes, is becoming increasingly central to CI/CD:
- Consistency across environments: Containers ensure that applications run the same way in development, testing, and production.
- Microservices architecture: Containers facilitate the deployment of microservices, allowing for more granular and frequent updates.
- Scalability: Container orchestration tools like Kubernetes enable easy scaling of applications and CI/CD infrastructure.
- Improved resource utilization: Containers allow for better use of hardware resources, reducing costs and improving efficiency.
(Source: Docker.docs, docs.docker.com)
Serverless CI/CD pipelines
Serverless computing is making its way into CI/CD:
- Reduced infrastructure management: Serverless CI/CD tools eliminate the need to maintain dedicated servers for builds and deployments.
- Auto-scaling: Serverless platforms can automatically scale resources based on workload, improving efficiency.
- Pay-per-use pricing: This model can significantly reduce costs for teams with variable build and deployment needs.
- Faster setup: Serverless CI/CD can be quicker to set up and easier to maintain than traditional server-based solutions.
Tools like AWS CodePipeline and Google Cloud Build are leading this trend, with more serverless CI server tools likely to emerge.
Enhanced security integration
Security is becoming an integral part of the CI/CD pipeline:
- Shift-left security: Security checks are moving earlier in the development process, with tools integrated directly into CI pipelines.
- Automated vulnerability scanning: Continuous scanning of code and dependencies for known vulnerabilities.
- Compliance as code: Automating compliance checks and audits as part of the CI/CD process.
- Secrets management: Improved tools and practices for managing sensitive information throughout the pipeline.
This trend towards “DevSecOps” ensures that security is not an afterthought but a core part of the development and deployment process.
Case Studies: Successful CI and CD Implementation
Company A: E-commerce Platform
Company A implemented CI and CD with Jenkins, reducing deployment time from days to hours and improving code quality. By leveraging Jenkins’ extensive plugin ecosystem, they were able to create a customized pipeline that fit their specific needs.
Company B: FinTech Startup
By adopting CI and CD practices and utilizing various CI server tools, Company B increased release frequency from monthly to weekly, gaining a competitive edge. They implemented a multi-tool approach, using Jenkins for their core pipeline and integrating specialized CI tools for specific tasks.
Conclusion
As we’ve explored throughout this guide, CI and CD are transformative practices that can significantly enhance software development processes. By automating builds, tests, and deployments, teams can achieve faster time to market, improved code quality, and increased collaboration between development and operations.
The choice of CI tools, whether it’s CI and CD with Jenkins or other CI server tools, plays a crucial role in the success of these practices. Each tool offers unique strengths, and the best choice depends on your specific project requirements and team preferences.
While implementing CI and CD comes with its challenges, the benefits far outweigh the initial hurdles. As the landscape continues to evolve with trends like AI-powered testing, containerization, and serverless architectures, staying informed and adaptable is key.
Whether you’re just starting with CI and CD or looking to optimize your existing pipelines, remember that the journey is iterative. By gradually incorporating best practices and leveraging tools like Jenkins and other CI tools, your team can build a robust CI/CD pipeline that drives efficiency, quality, and innovation in your software delivery process.
As you move forward, continue to evaluate and refine your CI/CD practices, stay open to new CI server tools and methodologies, and always keep the end goal in mind: delivering high-quality software to your users quickly and reliably.
Read our other article Mapping Your Business Success: Business and Financial Metrics to further improve your business.